Recently in patterns Category
Definition: The pattern is used to ensure that only one instance of an class is used. It offers a global point of access to this object. Before Java5 consider double checked locking to be thread-safe.
Example: Lets think of using only one connection to whatever throughout the program.
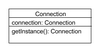
Connection:
public class Connection {
//volatile !!!!
private static volatile Connection connection;
//private Constructor
private Connection(){}
public static Connection getInstance(){
if (connection == null)//check first
{
synchronized(Connection.class) //one access per thread. sync only once
{
if (connection == null) //check again
return new Connection();
}
}
return connection;
}
}
Definition: The Command pattern defines the concept of encapsulating an action as an object and lets you parameterize clients with different requests, queue requests, log requests or undo operations.
Java Example: A classic example for an implementation of the command pattern is a remote control which switches the light on and off. An example for an command queue would be a disco-lighting system.
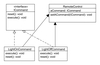
ICommand:
public interface ICommand {
public void execute();
public void reset();
}
LightOnCommand (LightOffCommand would simply print out "light off") :
public class LightOnCommand implements ICommand {
public void execute() {
System.out.println("light on");
}
public void reset() {@TODO}
}
Remote Control:
public class RemoteControl {
ICommand SwitchOn;
public void setCommand(ICommand aCommand)
{
SwitchOn= aCommand;
SwitchOn.execute();
}
}
RemoteControlTest:
public static void main(String [] args)
{
RemoteControl remote = new RemoteControl();
remote.setCommand(new LightOnCommand());
remote.setCommand(new LightOffCommand());
}
And this is an implementation for a command queue. Let´s go to the discotheque....
LightingSystem:
public class LightingSystem implements ICommand {
ICommand[] commands;
public LightingSystem (ICommand[] c)
{ commands = c; }
public void execute()
{
for (int i = 0; i < commands.length; i++) {
commands[i].execute();
}
}
public void reset() {
for (int i = 0; i < commands.length; i++) {
commands[i].reset();
}
}
}
And the test:
public static void main(String[] args) {
LightOnCommand on = new LightOnCommand ();
LightOffCommand off= new LightOffCommand ();
ICommand[] techno= {on,off,on,off,on,off,on,off};
LightingSystem strobo= new LightingSystem (techno);
strobo.execute();
}
Definition: The Observer Pattern defines a One-to-Many dependency between objects in a way that all dependent objects get automatically notified und updated, if the state of an object changes. Subjects or Observables notify Observers over an defined interface. Observers do not get notified in a particular order. Swing, JavaBeans and RMI use the Pattern often.
Java Example: A client registers to a newsletter and gets notified for every new news.
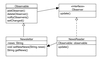
Subject:
public class Newsletter extends Observable
{
private String news;
public Newsletter(){}
public void setNewNews(String text)
{
this.news = text;
setChanged();
notifyObservers(this);
}
public String getNews()
{
return news;
}
Observer:
public class NewsReader implements Observer {
Observable observable;
public NewsReader(Observable ob)
{
this.observable = ob;
this.observable.addObserver(this);
}
public void update(Observable obs, Object obj) {
if (obs instanceof Newsletter) {
Newsletter newsletter = (Newsletter)obs;
System.out.println(newsletter.getNews());
}
}
}
Starting a new series to remember the good old gof design patterns. The STRATEGY Pattern will be my first entry.
Definition: "Define a family of algorithms, encapsulate each one, and make them interchangeable. Strategy lets the algorithm vary independently from the clients that use it." [Gamma]
Java Example: The client uses a capsuled family of algorithms. In this case we encapsulate a behaviour.

Client:
public abstract class Fighter {
FightingBehaviour fightingBehaviour;
public Fighter(){ };
public void fight(){fightingBehaviour.fight(); }
public void setFightingBehaviour(FightingBehaviour fb) {fightingBehaviour = fb;}
}
Client Implementations:
public class Boxer extends Fighter
{
public Boxer()
{
fightingBehaviour = new BoxingBehaviour();
}
}
Algorithm:
public interface FightingBehaviour {
public void fight();
}
Algorithm Implementation:
public class BoxingBehaviour implements FightingBehaviour {
public void fight() {
System.out.println("Raise fist!");
}
}
Test it:
Fighter fighter = new Boxer();
fighter.fight();
Thats basically it. It is now very easy to add new behaviours or fighters and change their algorithms.