Pattern Reminder: Command
Definition: The Command pattern defines the concept of encapsulating an action as an object and lets you parameterize clients with different requests, queue requests, log requests or undo operations.
Java Example: A classic example for an implementation of the command pattern is a remote control which switches the light on and off. An example for an command queue would be a disco-lighting system.
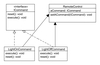
ICommand:
public interface ICommand {
public void execute();
public void reset();
}
LightOnCommand (LightOffCommand would simply print out "light off") :
public class LightOnCommand implements ICommand {
public void execute() {
System.out.println("light on");
}
public void reset() {@TODO}
}
Remote Control:
public class RemoteControl {
ICommand SwitchOn;
public void setCommand(ICommand aCommand)
{
SwitchOn= aCommand;
SwitchOn.execute();
}
}
RemoteControlTest:
public static void main(String [] args)
{
RemoteControl remote = new RemoteControl();
remote.setCommand(new LightOnCommand());
remote.setCommand(new LightOffCommand());
}
And this is an implementation for a command queue. Let´s go to the discotheque....
LightingSystem:
public class LightingSystem implements ICommand {
ICommand[] commands;
public LightingSystem (ICommand[] c)
{ commands = c; }
public void execute()
{
for (int i = 0; i < commands.length; i++) {
commands[i].execute();
}
}
public void reset() {
for (int i = 0; i < commands.length; i++) {
commands[i].reset();
}
}
}
And the test:
public static void main(String[] args) {
LightOnCommand on = new LightOnCommand ();
LightOffCommand off= new LightOffCommand ();
ICommand[] techno= {on,off,on,off,on,off,on,off};
LightingSystem strobo= new LightingSystem (techno);
strobo.execute();
}
0 TrackBacks
Listed below are links to blogs that reference this entry: Pattern Reminder: Command.
TrackBack URL for this entry: http://www.innoq.com/mt4/mt-tb.cgi/2464
Leave a comment